Node.js : hour 1
During half term, Em and the kids were off visiting family for a few days.
The Big Storm caused chaos to the trains, and so I hadn’t done my usual Monday commute to Shoreditch.
The combination meant that I found myself in the unusual position of having most of an evening to myself, so I wanted to see:
How much fun I could have with Node.js – in just an hour.
Node.js has had plenty of attention for some time, but hadn’t drawn me in until @adamyeats short talk at 12 Devs of Autumn. Ironically Adam’s talk followed @westleyknight in which he encouraged us not to feel like we had to learn everything….
Installing Node.js:
First step was to install node.js locally on my Mac for dev, and also I will be using a VPS (from Digital Ocean) for live.
Mac (local) using homebrew:
brew install node
Linux (VPS) Ubuntu:
https://mra.benseymour.comsudo apt-get install nodejs
(older ubuntu/distro’s may need guidance from here)
Node beginner
Node.js is a fairly popular topic currently and there are loads of greats resources, but I really liked the style, tone and pace of @manuelkiessling ’s http://www.nodebeginner.org/ (free to read online, or can purchase as part of a bundle for $10)
This stack overflow page also has a stack of great links: http://stackoverflow.com/questions/2353818/how-do-i-get-started-with-node-js
Hello world
The ubiquitous first program:
var http = require(“http”);
http.createServer(function(request, response){
response.writeHead(200,{“Content-Type”:”text/plain”});
response.write(“Hello World from Node.js”);
response.end();
}).listen(8888);
This file was called server1.js and so to run it:
node server1.js
and visit http://localhost:8888/server.js
Above “http” references one of the standard ‘modules’ that Node ships with.
For other modules, either write your own, or enjoy the gifts from this great community and use npm, which apparently it stands for ‘Node Packaged Modules’ (not node package manager) : https://npmjs.org/
nodemon
My first annoyance was stop-starting node whenever I made a code change. There appear to be a few options for this, but in my first test Remy Sharp’s (@rem) nodemon did exactly what I was looking for, so I didn’t look any further (why would i?!)
npm install nodemon -g
and from that point on instead of using:
node myfile.js
the following is used:
nodemon myfile.js
Whenever I now make code changes, in my terminal I saw:
28 Oct 21:20:37 – [nodemon] starting `node server1.js`
28 Oct 21:20:59 – [nodemon] restarting due to changes…
querystring
I wanted to extract the query string contents from the URL used for the request, and this is possible using standard functions:
var pathname = url.parse(request.url).pathname;
var query = url.parse(request.url).query;
console.log(“Request for ” + pathname + “ received with querystring: ” + query + ” “);
favicon
While I was experimenting with capturing querystring parameter, the console output was a little annoying as it kept showing 2 lines on favicon request whenever I viewed the page (this may be a quirk of Chrome), and so I used the following:
if (request.url === ‘/favicon.ico’) {
response.writeHead(200, {‘Content-Type’: ‘image/x-icon’} );
response.end();
//console.log(‘favicon requested’);
return; }
(I edited out the console log as this was what I was trying avoid – but clearly if you wanted to actually serve the favicon, the above needs further tweaking, but I’m leaving that for later)
Twitter – hello world
The more modern variant of the standard Hello World app, is to trigger a Twitter status update, for which I used the npm ‘node-twitter-api’ https://npmjs.org/package/node-twitter-api:
npm install node-twitter-api
You need to get your consumer key and consumer secret from
https://dev.twitter.com/ in the ‘manage your apps’ section. As my initial use will be local, and just for some initial basic status updates, I stored the access token and access token secret as variable:
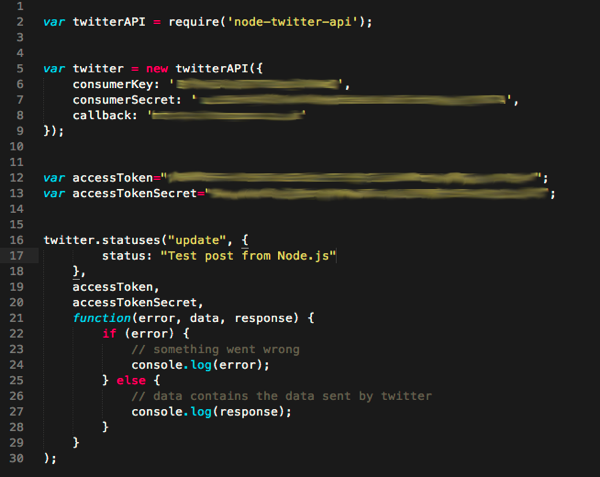
Upon running the code I got the following response:
data: ‘{“request”:”\/1.1\/statuses\/update.json”,”error”:”Read-only application cannot POST.”}’
So I updated the application type setting on dev.twitter.com:
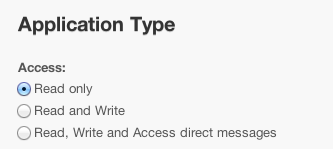
– and then ensure you recreate your access token. Run the code, and hey presto:
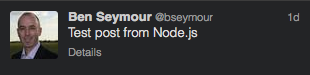
Twilio – hello world
Another favourite hello world is to use the wonderful Twilio API to send an SMS to my phone. My time was nearly up, but I gave it a go.
npm install twilio
(I’m becoming rather fond of npm, and it seems that while I had been avoiding node.js, a lot of interesting folks and companies have developed their node modules)
I grabbed my Twilio account SID and Auth Token, and using the code example from here: https://www.twilio.com/blog/2013/03/introducing-the-twilio-module-for-node-js.html :
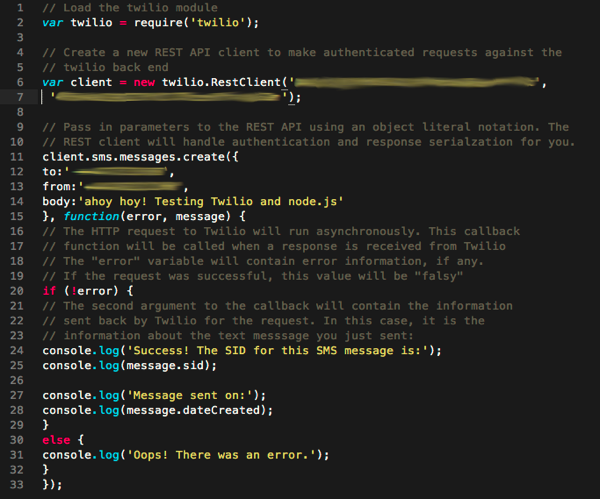
and moments later my mobile received:
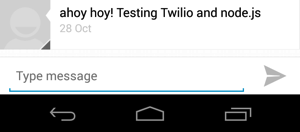
All in all, the hour was superb. I’ve barely scratched the surface of Node.js, but it already feels like once of those programming languages that is going to be thoroughly enjoyable to use….. somehow having a very low coefficient of friction.
Next time, I was to explore which other services and products I use have npm’s : fitbit, google docs,….